If you have user-generated content in your web application, chances are you have to deal with strings containing emojis. Since emojis are stored as Unicode, chances are that we want to detect these in our code and then render them accordingly. This article discusses how we can check if a string contains emojis in JavaScript.
A Unicode representation of emojis looks something like this:
'\u{1F60A}' // "π"
'\u{1F42A}' // "πͺ"
JavaScriptAnd JavaScript regular expressions have a Unicode mode now, so we can use Unicode property escapes to check for various things like emojis and currency symbols.
const emojiRegex = /\p{Emoji}/u;
emojiRegex.test('π'); // true
JavaScriptAnd it has good browser support as well:
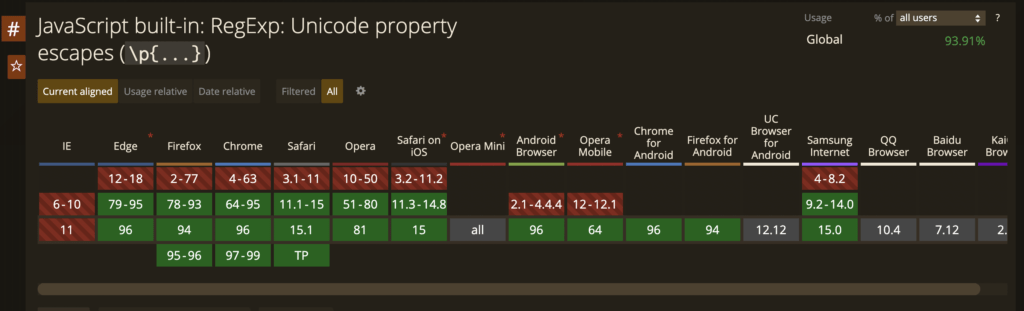
And if we wanted to do a replace, we can do:
'replacing emojis ππͺ'.replaceAll(/\p{Emoji}/ug, '');
// 'replacing emojis'
JavaScriptThe “g” flag was used to replace all emojis.
It is worth noting that some emojis are a combination of multiple emojis (or code points). So, this is not a fail-safe approach and there can be some more nuances:
"π―π΅".replaceAll(/\p{Emoji}/gu, '-'); // '--'
"ππΏ".replaceAll(/\p{Emoji}/gu, '-'); // '--'
"π¨βπ©βπ§βπ¦".replaceAll(/\p{Emoji}/gu, '-'); // '----'
JavaScriptThe country flags are a combination of regional symbol indicator letters” (π― + π΅), the emoji followed by a skin tone modifier is again a combination, and the family one is a combination of 4 different emojis.