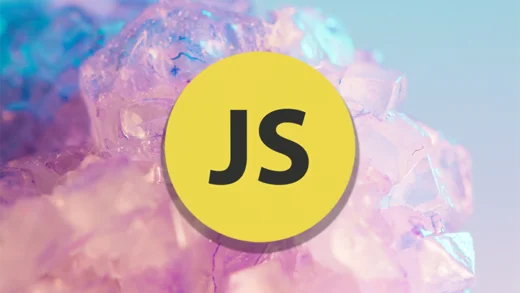
JavaScript: fix “SyntaxError: cannot use import statement outside a module”
I recently was working with a third-party library. I ran into the error “Uncaught SyntaxError: cannot use import statement outside a module” when I tried to import a function from the package. Let us look into why it occurred and how I fixed it. Why is this happening? The error...