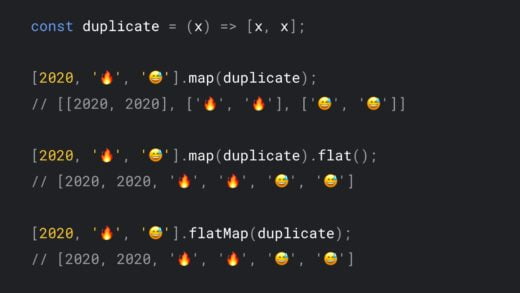
Flatten Arrays in Vanilla JavaScript with flat() and flatMap()
ES2019 introduced two methods on the array prototype that would make life so much simpler for developers. These are flat() and flatmap() which help in flattening arrays. Array.prototype.flat() As the name suggests, the flat() method returns a new array with elements of the subarray flattened into it, that is the...